Use Python to update a spreadsheet
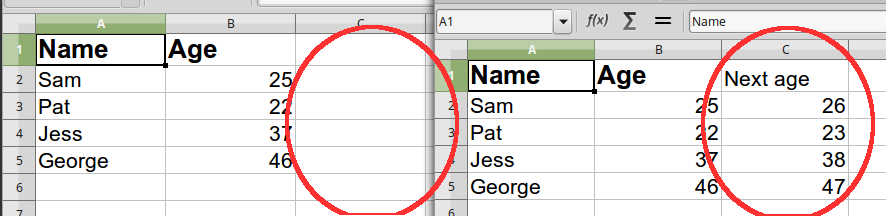
How would you like to grab a share price daily and store it in a spreadsheet? Or add a new column to dozens of spreadsheets – automatically?
Python is a simple but powerful language, and comes with a wealth of libraries. Its openpyxl library lets you easily open a spreadsheet and make some changes.
Here is an example which adds a new column (“Next age”) to all spreadsheets in the source_folder. The left side of the image above shows an original spreadsheet. The Python script opens this, adds a new column (Next age), then saves it to the target_folder. The right side of the image shows the result
Here is the annotated code. You can find the raw code at the GitHub repository
Before installing openpyxl, to keep your Python version(s) clean, you may want to set up a virtual environment first
To install openpyxl: pip install openpyxl
1. import openpyxl
2. import os
3. for name in os.listdir('source_files'):
4. workbook = openpyxl.load_workbook(filename='source_files/' + name)
5. sheet = workbook['Sheet1']
6. sheet['C1'].value = 'Next age'
7. for row in range(2, 100):
8. if sheet[f'B{row}'].value:
9. sheet[f'C{row}'].value = sheet[f'B{row}'].value + 1
10. workbook.save(filename='target_files/' + name)
1. import openpyxl Load the openpyxl library.
2. import os Load the os library. We will use this list the files in a folder
3. for name in os.listdir(‘source_files’): For each file in our ‘source_files’ folder. Note that this includes all files, regardless of whether it is a spreadsheet or not
4. workbook = openpyxl.load_workbook(filename=’source_files/’ + name) Open the workbook
5. sheet = workbook[‘Sheet1’] Take the worksheet called ‘Sheet1’
6. sheet[‘C1’].value = ‘Next age’ Enter something in cell C1
7. for row in range(2, 100): For rows 2 – 99 (Python stops just before reaching 100), do the following:
8. if sheet[f’B{row}’].value: If cell B2, B3, B4, etc is not empty, do the following:
9. sheet[f’C{row}’].value = sheet[f’B{row}’].value + 1 Take the age from column B, add one to it and store in the cell to the right, i.e. in column C
10. workbook.save(filename=’target_files/’ + name) Save the updated workbook to the target_files folder, using the same name