Take out your turtle for a recursive walk
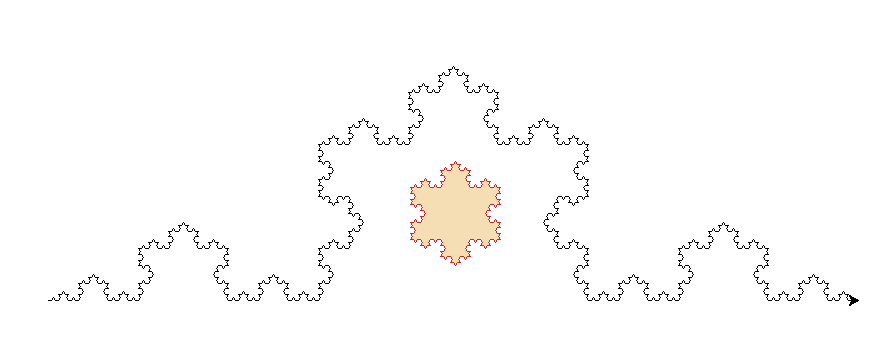
Python is a simple but powerful language, and comes with a wealth of libraries. It took just 10 lines of code and the Turtle library to create the black line in the image above.
Turtle graphics is popular for introducing programming, particularly to children. It shows a ‘turtle’ (triangle) which moves over the ‘paper’ whilst drawing a line. It is a fun way to try out some programming ideas.
The idea of recursion is that you break a large task down into a set of smaller tasks. In this case, to draw a long line, we give it a bit of flourish by drawing 4 shorter lines instead, like this:
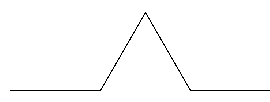
Each of those shorter lines is broken down into 4 even shorter lines, like this:
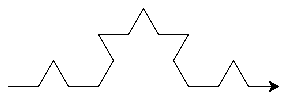
And again:
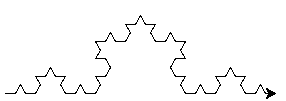
And once more to get the final picture (above)
Here is the code for the black line. You can find the raw code at the GitHub repository
1. import turtle Import the turtle library
2. def line(length): Create a new function which draws a line of length ‘length’. However, it may make the line a bit wiggly
3. if length <= 5: If it is a short line, just draw it
4. turtle.forward(length)
Move the turtle
5. return Exit this function
6. for angle in (60, -120, 60, 0): Go forward (straight or wiggly) for one-third of the required distance, then turn left 60 degrees. Repeat for right 120 degrees, left 60 degrees, and straight again. By the end of this the turtle will be pointing in exactly the same direction as before.
7. line(length / 3) Draw the line (straight or wiggly)
8. turtle.left(angle) Turn the requested angle
9. line(810) Ask the ‘line’ function to draw a line of length 810. The recursive pattern will make it very wiggly
10. turtle.done() Wait until the user closes the window. Without this the window would close as soon as the pattern has been drawn
To draw the coloured snowflake (see the image at the start of this article), use the following code:
Note: the “turtle.color()” call sets the line colour and the colour used to fill in the final shape
import turtle
def line(length):
if length <= 5:
turtle.forward(length)
return
for angle in (60, -120, 60, 0):
line(length / 3)
turtle.left(angle)
turtle.begin_fill()
turtle.color('firebrick3', 'wheat')
for _ in range(3):
line(90)
turtle.right(120)
turtle.end_fill()
turtle.done()